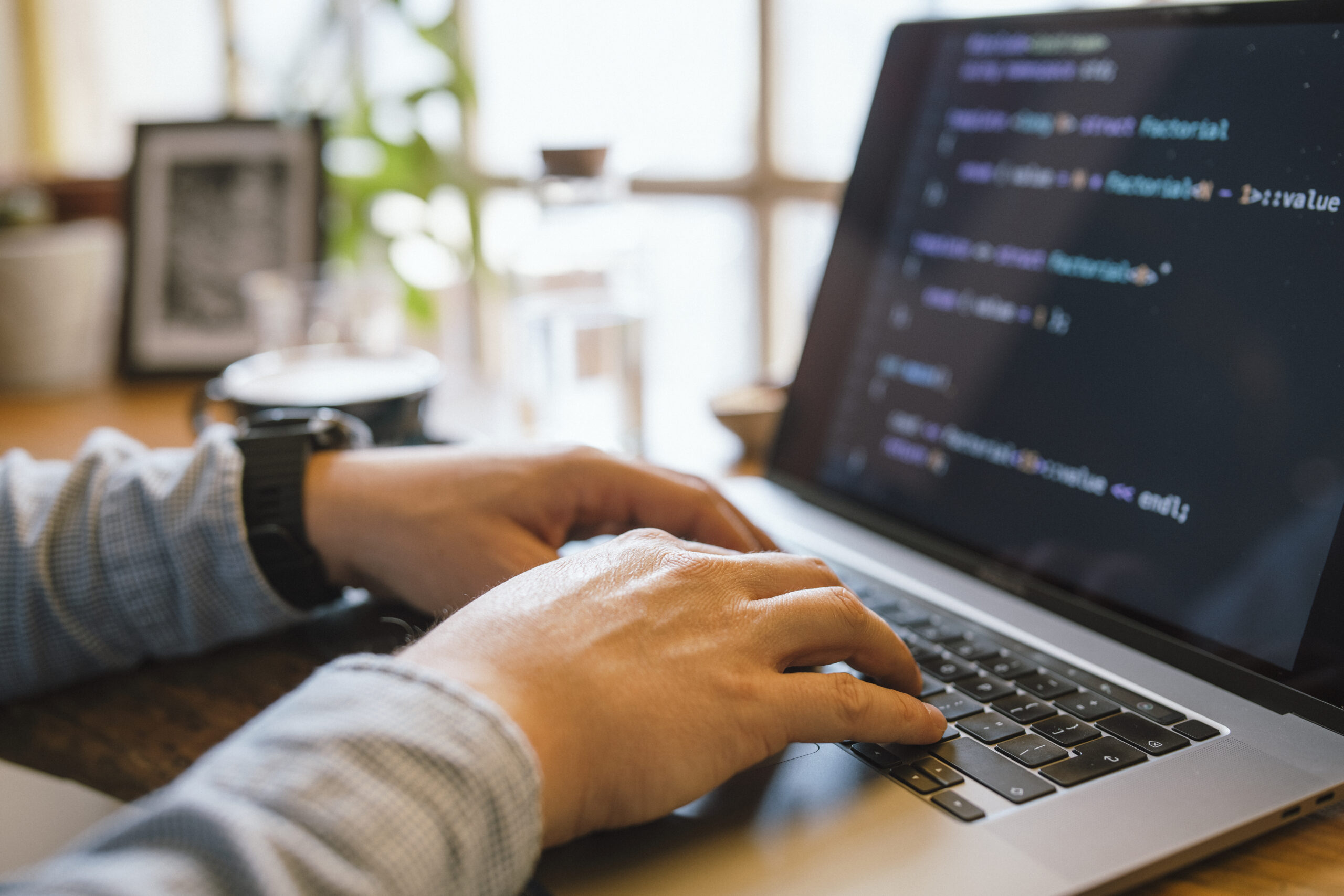
Debugging is Probably the most necessary — nonetheless often ignored — capabilities in a very developer’s toolkit. It isn't really pretty much repairing damaged code; it’s about knowledge how and why matters go Completely wrong, and learning to think methodically to resolve troubles successfully. Irrespective of whether you are a starter or simply a seasoned developer, sharpening your debugging abilities can conserve hours of aggravation and significantly enhance your productivity. Listed below are numerous techniques to aid developers amount up their debugging activity by me, Gustavo Woltmann.
Master Your Applications
On the list of fastest approaches developers can elevate their debugging skills is by mastering the applications they use every day. While crafting code is just one Section of advancement, understanding the best way to interact with it correctly through execution is equally important. Fashionable enhancement environments appear equipped with impressive debugging capabilities — but numerous builders only scratch the surface area of what these equipment can perform.
Acquire, as an example, an Built-in Growth Atmosphere (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These instruments let you established breakpoints, inspect the value of variables at runtime, move by way of code line by line, and in some cases modify code around the fly. When applied appropriately, they let you observe particularly how your code behaves in the course of execution, that is a must have for tracking down elusive bugs.
Browser developer instruments, like Chrome DevTools, are indispensable for entrance-end builders. They let you inspect the DOM, watch network requests, look at real-time effectiveness metrics, and debug JavaScript in the browser. Mastering the console, resources, and network tabs can change disheartening UI concerns into workable responsibilities.
For backend or process-level developers, equipment like GDB (GNU Debugger), Valgrind, or LLDB give deep Manage in excess of functioning procedures and memory administration. Studying these equipment could possibly have a steeper learning curve but pays off when debugging efficiency difficulties, memory leaks, or segmentation faults.
Past your IDE or debugger, develop into snug with version Manage programs like Git to be aware of code background, uncover the precise minute bugs were being released, and isolate problematic changes.
In the end, mastering your instruments usually means going past default settings and shortcuts — it’s about building an intimate understanding of your enhancement environment to ensure that when problems come up, you’re not misplaced at midnight. The higher you recognize your instruments, the greater time it is possible to commit fixing the actual dilemma in lieu of fumbling by way of the method.
Reproduce the trouble
Just about the most vital — and often neglected — methods in successful debugging is reproducing the trouble. Prior to leaping into your code or building guesses, builders will need to make a constant environment or state of affairs wherever the bug reliably appears. With no reproducibility, fixing a bug becomes a activity of probability, usually leading to squandered time and fragile code improvements.
Step one in reproducing a problem is accumulating as much context as possible. Check with inquiries like: What actions triggered The problem? Which atmosphere was it in — enhancement, staging, or creation? Are there any logs, screenshots, or error messages? The greater detail you have, the much easier it gets to be to isolate the precise situations less than which the bug happens.
Once you’ve gathered adequate information and facts, try and recreate the problem in your neighborhood environment. This might suggest inputting a similar info, simulating identical consumer interactions, or mimicking process states. If the issue appears intermittently, contemplate crafting automated assessments that replicate the sting instances or condition transitions associated. These exams not just enable expose the issue and also prevent regressions Later on.
From time to time, The difficulty can be atmosphere-distinct — it'd happen only on specific running units, browsers, or beneath unique configurations. Using resources like virtual devices, containerization (e.g., Docker), or cross-browser tests platforms is usually instrumental in replicating such bugs.
Reproducing the situation isn’t simply a step — it’s a state of mind. It needs endurance, observation, and also a methodical solution. But once you can persistently recreate the bug, you happen to be by now midway to correcting it. Which has a reproducible state of affairs, you can use your debugging equipment far more properly, take a look at probable fixes safely and securely, and converse additional Plainly with your team or users. It turns an summary criticism right into a concrete problem — and that’s exactly where builders thrive.
Go through and Realize the Error Messages
Mistake messages will often be the most beneficial clues a developer has when some thing goes Incorrect. Rather than looking at them as disheartening interruptions, builders need to find out to treat error messages as immediate communications with the technique. They usually tell you exactly what took place, the place it took place, and at times even why it happened — if you know the way to interpret them.
Start off by reading through the message thoroughly and in total. Numerous builders, particularly when below time pressure, look at the 1st line and quickly start out generating assumptions. But deeper during the error stack or logs might lie the legitimate root lead to. Don’t just copy and paste mistake messages into serps — examine and realize them first.
Split the error down into sections. Could it be a syntax error, a runtime exception, or perhaps a logic mistake? Does it issue to a certain file and line quantity? What module or functionality induced it? These concerns can guideline your investigation and position you toward the liable code.
It’s also valuable to know the terminology of your programming language or framework you’re making use of. Mistake messages in languages like Python, JavaScript, or Java often comply with predictable styles, and Understanding to acknowledge these can dramatically hasten your debugging procedure.
Some errors are obscure or generic, As well as in These scenarios, it’s essential to look at the context wherein the error transpired. Verify relevant log entries, enter values, and recent adjustments from the codebase.
Don’t overlook compiler or linter warnings both. These generally precede greater difficulties and supply hints about possible bugs.
Eventually, mistake messages are usually not your enemies—they’re your guides. Studying to interpret them appropriately turns chaos into clarity, serving to you pinpoint problems more rapidly, lower debugging time, and turn into a extra efficient and assured developer.
Use Logging Correctly
Logging is One of the more effective equipment in a very developer’s debugging toolkit. When made use of effectively, it provides genuine-time insights into how an application behaves, aiding you recognize what’s happening under the hood without needing to pause execution or step with the code line by line.
A great logging method begins with realizing what to log and at what amount. Popular logging concentrations involve DEBUG, Facts, Alert, ERROR, and FATAL. Use DEBUG for comprehensive diagnostic info for the duration of progress, Details for standard activities (like productive begin-ups), WARN for probable difficulties that don’t crack the applying, ERROR for real problems, and Lethal if the program can’t carry on.
Avoid flooding your logs with excessive or irrelevant details. An excessive amount logging can obscure critical messages and slow down your procedure. Center on essential occasions, point out variations, enter/output values, and critical conclusion factors inside your code.
Structure your log messages Obviously and consistently. Include things like context, including timestamps, request IDs, and performance names, so it’s simpler to trace issues in dispersed methods or multi-threaded environments. Structured logging (e.g., JSON logs) will make it even simpler to parse and filter logs programmatically.
Throughout debugging, logs Enable you to observe how variables evolve, what circumstances are achieved, and what branches of logic are executed—all without having halting This system. They’re Specifically important in creation environments where by stepping by means of code isn’t probable.
On top of that, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that aid log rotation, filtering, and integration with monitoring dashboards.
In the long run, wise logging is about stability and clarity. That has a nicely-considered-out logging strategy, you could reduce the time it will require to identify problems, get further visibility into your applications, and Enhance the Over-all maintainability and dependability of your respective code.
Think Like a Detective
Debugging is not simply a technological task—it's a kind of investigation. To proficiently identify and deal with bugs, builders must method the method just like a detective resolving a secret. This mindset assists stop working elaborate issues into manageable elements and comply with clues logically to uncover the basis induce.
Start by gathering evidence. Consider the indicators of the condition: mistake messages, incorrect output, or performance issues. Just like a detective surveys a crime scene, gather as much related info as you may without the need of leaping to conclusions. Use logs, take a look at scenarios, and person stories to piece jointly a transparent image of what’s taking place.
Upcoming, sort hypotheses. Question by yourself: What may be triggering this conduct? Have any adjustments lately been produced to the codebase? Has this concern occurred before less than very similar situation? The aim is always to narrow down alternatives and establish prospective culprits.
Then, examination your theories systematically. Make an effort to recreate the issue inside of a managed surroundings. If you suspect a selected operate or component, isolate it and validate if The difficulty persists. Just like a detective conducting interviews, inquire your code thoughts and Allow the results guide you closer to the reality.
Pay out shut consideration to smaller specifics. Bugs often cover within the the very least anticipated sites—just like a missing semicolon, an off-by-a person error, or perhaps a race condition. Be comprehensive and patient, resisting the urge to patch The problem without thoroughly knowing it. Non permanent fixes could disguise the actual dilemma, just for it to resurface later.
And lastly, preserve notes on what you experimented with and learned. Equally as detectives log their investigations, documenting your debugging method can preserve time for future troubles and assistance Other individuals recognize your reasoning.
By wondering like a detective, developers can sharpen their analytical competencies, solution difficulties methodically, and develop into more practical at uncovering hidden concerns in advanced systems.
Compose Assessments
Crafting checks is one of the simplest ways to boost your debugging capabilities and read more In general development efficiency. Exams not merely support capture bugs early and also serve as a safety net that gives you self-assurance when generating improvements towards your codebase. A well-tested software is much easier to debug because it permits you to pinpoint just the place and when a challenge happens.
Begin with unit exams, which give attention to specific features or modules. These tiny, isolated exams can speedily expose no matter if a certain piece of logic is Functioning as anticipated. When a test fails, you immediately know where to glimpse, noticeably lessening the time used debugging. Device exams are Specifically helpful for catching regression bugs—issues that reappear just after Earlier currently being set.
Subsequent, combine integration tests and end-to-conclusion exams into your workflow. These assist ensure that many areas of your application do the job jointly easily. They’re particularly handy for catching bugs that manifest in intricate methods with various elements or solutions interacting. If a little something breaks, your exams can tell you which Section of the pipeline failed and underneath what situations.
Crafting checks also forces you to Assume critically about your code. To check a characteristic properly, you may need to know its inputs, predicted outputs, and edge instances. This standard of comprehending The natural way potential customers to better code framework and fewer bugs.
When debugging a problem, crafting a failing check that reproduces the bug is often a powerful initial step. As soon as the check fails persistently, you can deal with fixing the bug and look at your exam pass when The problem is solved. This approach ensures that the exact same bug doesn’t return in the future.
In brief, producing checks turns debugging from the discouraging guessing game into a structured and predictable method—serving to you capture more bugs, more quickly plus much more reliably.
Choose Breaks
When debugging a tricky problem, it’s effortless to be immersed in the problem—watching your display screen for several hours, seeking solution following Remedy. But The most underrated debugging instruments is solely stepping absent. Having breaks allows you reset your intellect, cut down frustration, and sometimes see The problem from a new perspective.
When you're as well close to the code for as well lengthy, cognitive fatigue sets in. You may begin overlooking obvious errors or misreading code that you wrote just hrs earlier. Within this state, your Mind will become a lot less successful at dilemma-fixing. A short wander, a espresso split, and even switching to a special activity for 10–quarter-hour can refresh your concentration. A lot of developers report finding the foundation of a challenge once they've taken time for you to disconnect, permitting their subconscious get the job done while in the track record.
Breaks also help reduce burnout, especially all through more time debugging sessions. Sitting down in front of a screen, mentally trapped, is not merely unproductive but also draining. Stepping absent permits you to return with renewed Power in addition to a clearer frame of mind. You could instantly notice a lacking semicolon, a logic flaw, or perhaps a misplaced variable that eluded you right before.
In case you’re trapped, a superb rule of thumb will be to set a timer—debug actively for forty five–60 minutes, then take a five–10 moment break. Use that point to move all over, stretch, or do a thing unrelated to code. It might sense counterintuitive, Particularly underneath tight deadlines, but it surely really brings about quicker and simpler debugging in the long run.
In a nutshell, having breaks is just not an indication of weakness—it’s a wise system. It provides your Mind House to breathe, improves your viewpoint, and allows you avoid the tunnel vision That usually blocks your development. Debugging is really a mental puzzle, and relaxation is part of fixing it.
Study From Each Bug
Each and every bug you face is a lot more than just a temporary setback—It truly is a possibility to grow as being a developer. No matter if it’s a syntax mistake, a logic flaw, or a deep architectural problem, each can instruct you something beneficial in case you make the effort to replicate and review what went Incorrect.
Commence by asking oneself a number of critical thoughts as soon as the bug is fixed: What caused it? Why did it go unnoticed? Could it happen to be caught earlier with greater techniques like device screening, code testimonials, or logging? The solutions typically reveal blind spots within your workflow or knowing and allow you to Construct more powerful coding behavior shifting forward.
Documenting bugs can also be an excellent habit. Preserve a developer journal or preserve a log where you note down bugs you’ve encountered, the way you solved them, and That which you uncovered. After a while, you’ll start to see patterns—recurring issues or popular faults—which you could proactively keep away from.
In group environments, sharing what you've acquired from the bug along with your peers may be especially impressive. No matter if it’s by way of a Slack message, a brief compose-up, or A fast know-how-sharing session, aiding others steer clear of the identical problem boosts workforce effectiveness and cultivates a much better Mastering culture.
Extra importantly, viewing bugs as classes shifts your attitude from frustration to curiosity. In place of dreading bugs, you’ll commence appreciating them as critical areas of your development journey. In spite of everything, a number of the most effective developers are usually not the ones who produce ideal code, but individuals that constantly master from their blunders.
Eventually, Every bug you fix adds a completely new layer in your talent set. So up coming time you squash a bug, have a moment to reflect—you’ll appear absent a smarter, much more able developer thanks to it.
Conclusion
Strengthening your debugging competencies will take time, exercise, and tolerance — however the payoff is big. It tends to make you a more successful, self-assured, and capable developer. The following time you happen to be knee-deep in a very mysterious bug, remember: debugging isn’t a chore — it’s an opportunity to become superior at Anything you do.